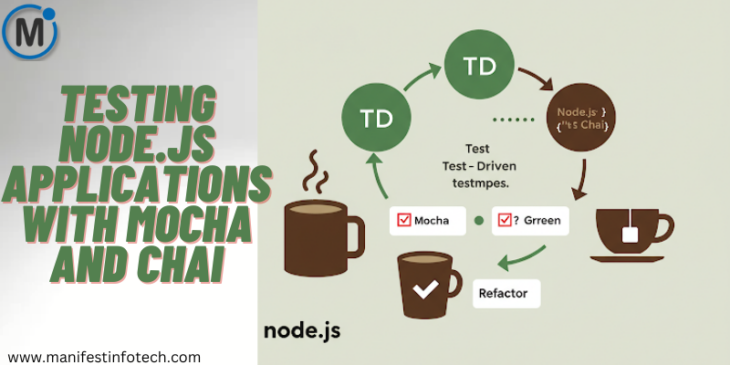
An Introduction to Test-Driven Development (TDD)
In modern software development, testing is essential to ensure reliability, maintainability, and confidence in your code. When it comes to Node.js, two popular tools stand out for unit testing: Mocha and Chai. By combining these tools with a Test-Driven Development (TDD) approach, you can streamline your development process and produce high-quality applications.
What Is TDD?
Test-Driven Development (TDD) is a methodology where developers write tests before writing the actual code. The process follows a cycle often described as Red-Green-Refactor:
Red: Write a test that fails initially (because the functionality isn’t implemented yet).
Green: Write just enough code to make the test pass.
Refactor: Clean up and optimize the code, ensuring all tests still pass.
This approach ensures that every new feature or change is validated against a set of predefined requirements. TDD helps catch bugs early, promotes clean architecture, and instills confidence in refactoring efforts.
Introducing Mocha
Mocha is a feature-rich JavaScript test framework running on Node.js, known for its simplicity and flexibility. Key benefits of using Mocha include:
Easy Setup: Installing Mocha is straightforward—just run npm install –save-dev mocha.
Descriptive Syntax: Mocha uses describe and it blocks, making tests highly readable.
Asynchronous Testing: Support for async/await or callbacks helps handle asynchronous code gracefully.
Here’s a basic example of a Mocha test structure:
javascript
Copy
Edit
const assert = require(‘assert’);
describe(‘Array’, function() {
it(‘should return -1 when the value is not present’, function() {
assert.strictEqual([1, 2, 3].indexOf(4), -1);
});
});
In this snippet, the describe block groups related tests, and the it block contains a single test scenario.
Introducing Chai
While Mocha provides the testing framework, Chai is an assertion library that makes your tests more expressive. Chai supports three assertion styles:
Assert (Node’s built-in style)
Expect (human-readable BDD style)
Should (prototype-based BDD style)
Using Chai’s expect style often looks like this:
javascript
Copy
Edit
const { expect } = require(‘chai’);
describe(‘Mathematical Operations’, () => {
it(‘should correctly add two numbers’, () => {
const result = 2 + 3;
expect(result).to.equal(5);
});
});
The expect(result).to.equal(5); syntax is more descriptive and aligns well with TDD principles.
Setting Up Your Project
Initialize Node.js: Run npm init -y to create a package.json.
Install Mocha & Chai:
bash
Copy
Edit
npm install –save-dev mocha chai
Configure Scripts: In package.json, add a test script:
json
Copy
Edit
“scripts”: {
“test”: “mocha”
}
Create Test Folder: Store your tests in a test directory.
Write Tests First: Following TDD, write failing tests before implementing the functionality.
Benefits of Mocha + Chai + TDD
Confidence: TDD ensures your code does what it’s supposed to do from the start.
Fewer Bugs: Writing tests before coding catches issues early.
Better Design: TDD often leads to cleaner, more modular code.
Easy Refactoring: With a solid test suite, you can refactor without fear of breaking existing functionality.
Conclusion
By using Mocha and Chai in a TDD workflow, you can build robust Node.js applications that are well-structured, maintainable, and bug-free. The Red-Green-Refactor cycle ensures you always code with purpose and validate your logic step by step. If you haven’t started testing yet, give Mocha and Chai a try—they’re easy to learn, powerful, and fit seamlessly into the Node.js ecosystem.
#NodeJS #Mocha #Chai #TDD #UnitTesting #TestDrivenDevelopment #JavaScript #WebDevelopment #SoftwareTesting #Backend