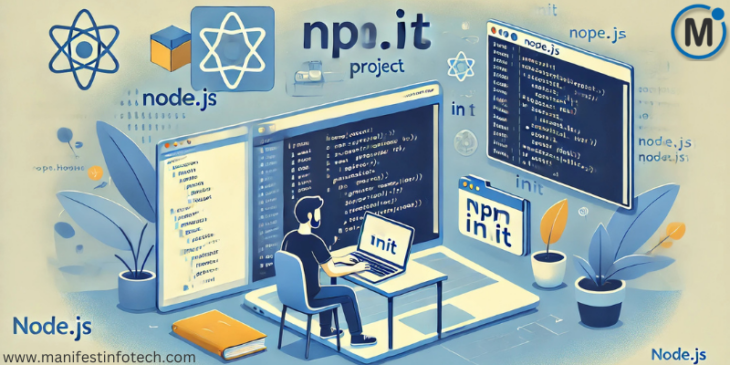
Starting a Node.js project for the first time can feel daunting, but with the right steps, you can have a functional project up and running in no time. Node.js is an incredibly powerful platform, and setting up your first project is an excellent way to familiarize yourself with its core features and workflows.
Step 1: Install Node.js
Before you can start, you need to have Node.js installed on your system. Visit the official Node.js website and download the latest Long-Term Support (LTS) version for your operating system. The installation process will also include NPM (Node Package Manager), a vital tool for managing packages and dependencies.
To verify the installation, open your terminal or command prompt and type:
node -v npm -v
These commands will display the installed versions of Node.js and NPM.
Step 2: Create Your Project Directory
Next, create a directory for your Node.js project. This will serve as the workspace where all your files and dependencies will be stored.
mkdir my-first-node-project
cd my-first-node-project
Replace my-first-node-project with a name of your choice.
Step 3: Initialize Your Project
Within your project directory, initialize a new Node.js project using the following command:
npm init
This will start a step-by-step process where you’ll be asked to provide information about your project, such as its name, version, description, entry point, and author. If you want to skip the prompts, you can use:
npm init -y
This will create a package.json file with default values. The package.json file serves as the blueprint for your project, listing dependencies, scripts, and other metadata.
Step 4: Install Dependencies
Node.js projects often rely on external libraries or frameworks. To add a dependency, use:
npm install
For example, to install Express, a popular Node.js framework:
npm install express
This command will add the package to your node_modules folder and update your package.json file accordingly.
Step 5: Create the Entry File
Most Node.js projects start with an entry file, typically named index.js . Create this file in your project directory:
touch index.js
Open the file in your preferred code editor and add the following code to test your setup:
console.log(“Hello, Node.js!”);
Step 6: Run Your Application
To execute your Node.js application, return to your terminal and type:
node index.js
You should see the output:
Hello, Node.js!
This confirms that Node.js is correctly installed and your project is set up.
Step 7: Add a Simple HTTP Server
Take it a step further by creating a simple HTTP server. Replace the code in index.js with:
const http = require(‘http’); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader(‘Content-Type’, ‘text/plain’); res.end(‘Hello, World!n’); }); const PORT = 3000; server.listen(PORT, () => { console.log(`Server running at http://localhost:${PORT}/`); });
Run the file again with:
node index.js
Visit http://localhost:3000 in your browser, and you should see “Hello, World!”
Conclusion
Setting up your first Node.js project is an exciting milestone. By following these steps, you’ve learned how to install Node.js, initialize a project, manage dependencies, and create a basic application. As you continue exploring Node.js, you’ll discover its vast capabilities for building scalable and efficient server-side applications. Happy coding!
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#Nodejs #JavaScript #WebDevelopment #ServerSide #RealTimeApps #EventDriven #Asynchronous #NPM #HighPerformance #Scalability #Microservices #IoT #BackendDevelopment #FullStack #NodejsCommunity #TechInnovation #SoftwareDevelopment #OpenSource #DeveloperTools #CrossPlatform #AppDevelopment #NodejsTutorial