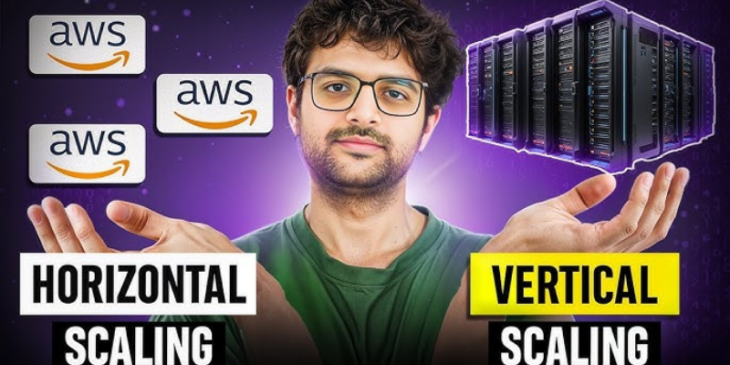
Node.js is a powerful JavaScript runtime that enables developers to build high-performance, scalable applications. As your application grows, handling increased traffic efficiently becomes crucial. Scaling ensures your Node.js app maintains speed, reliability, and performance under load. In this blog, we’ll explore horizontal and vertical scaling techniques and key strategies like load balancing, clustering, and microservices.
Understanding Scaling in Node.js
Scaling refers to increasing an application’s capacity to handle more requests efficiently. There are two primary ways to scale a Node.js application:
Vertical Scaling – Increasing the resources (CPU, RAM) of a single server.
Horizontal Scaling – Adding more servers or instances to distribute the load.
Each approach has its advantages and best-use scenarios.
Vertical Scaling in Node.js
Vertical scaling, also known as “scaling up,” involves upgrading the hardware of a single server to improve performance. This method is straightforward but has limitations:
Advantages:
Quick and easy to implement.
No need to modify the application architecture.
Disadvantages:
Limited by hardware constraints.
More expensive as high-end servers cost more.
Horizontal Scaling in Node.js
Horizontal scaling, or “scaling out,” involves distributing the application load across multiple servers. This approach provides better redundancy, fault tolerance, and flexibility.
Advantages:
Scales infinitely by adding more servers.
Provides high availability and fault tolerance.
Reduces the impact of server failures.
Disadvantages:
More complex to implement and manage.
Requires load balancing and distributed architecture.
Techniques for Scaling Node.js Applications
1.Load Balancing
A load balancer distributes incoming requests across multiple instances of the application, preventing any single server from becoming overwhelmed. Popular load balancers include:
NGINX
HAProxy
AWS Elastic Load Balancer (ELB)
2. Clustering
Node.js has a built-in cluster module that allows multiple processes to run on a single machine, utilizing multiple CPU cores.
Example: Using the Cluster Module
const cluster = require(‘cluster’);
const http = require(‘http’);
const os = require(‘os’);
if (cluster.isMaster) {
const numCPUs = os.cpus().length;
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end(‘Hello from Node.js Cluster!’);
}).listen(8000);
}
This approach utilizes all CPU cores to improve efficiency.
3. Microservices Architecture
Instead of building a monolithic Node.js application, breaking it down into smaller microservices allows independent scaling of different components. Technologies like Docker, Kubernetes, and API gateways help manage microservices efficiently.
4. Caching
Using caching techniques with Redis or Memcached reduces database queries and improves performance.
5. Containerization & Orchestration
Using Docker and Kubernetes helps automate deployment and scaling in cloud environments like AWS, Azure, and Google Cloud.
Conclusion
Scaling Node.js applications is essential to ensure performance and reliability as user demand grows. While vertical scaling is easy, horizontal scaling offers better long-term benefits. Implementing load balancing, clustering, microservices, and caching can significantly enhance scalability. By choosing the right strategy, you can build robust, scalable, and efficient applications.
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#NodeJS #WebDevelopment #Scalability #LoadBalancing #Microservices #CloudComputing #ScalingNodeJS