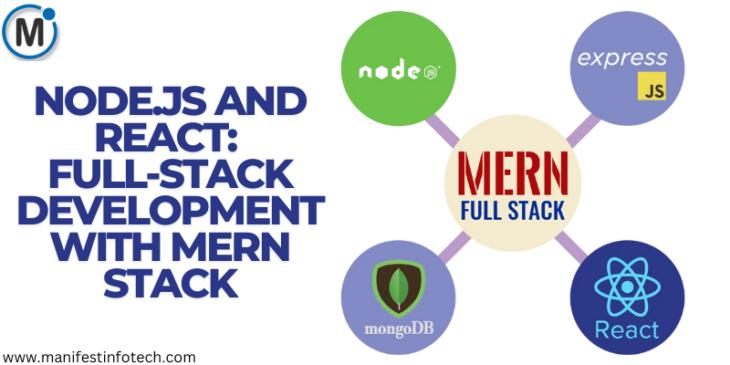
The MERN stack (MongoDB, Express.js, React.js, and Node.js) is one of the most popular full-stack development technologies. It enables developers to build modern web applications using JavaScript for both frontend and backend. This guide will walk you through the fundamentals of developing a full-stack application using the MERN stack.
What is the MERN Stack?
MERN is a combination of four powerful technologies:
MongoDB: A NoSQL database for storing application data in JSON-like documents.
Express.js: A lightweight and fast Node.js framework for handling HTTP requests and APIs.
React.js: A JavaScript library for building dynamic user interfaces.
Node.js: A runtime environment for executing JavaScript on the server.
By using JavaScript throughout the stack, MERN ensures seamless communication between frontend and backend components, making development efficient and scalable.
Setting Up the MERN Stack
To build a MERN application, follow these steps:
1. Install Node.js and MongoDB
Ensure you have Node.js and MongoDB installed on your system. You can check by running:
node -v
mongo –version
2. Create the Backend with Node.js and Express
Initialize a new Node.js project:
mkdir mern-app && cd mern-app
npm init -y
Install Express and other dependencies:
npm install express mongoose cors dotenv
Create a basic Express server (server.js):
const express = require(‘express’);
const mongoose = require(‘mongoose’);
const cors = require(‘cors’);
require(‘dotenv’).config();
const app = express();
app.use(express.json());
app.use(cors());
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true
}).then(() => console.log(‘MongoDB Connected’)).catch(err => console.log(err));
app.listen(5000, () => console.log(‘Server running on port 5000’));
3. Define the Database Schema
Create a model file (models/User.js):
const mongoose = require(‘mongoose’);
const UserSchema = new mongoose.Schema({
name: String,
email: String,
password: String
});
module.exports = mongoose.model(‘User’, UserSchema);
4. Set Up React Frontend
Create a React app:
npx create-react-app client
cd client
npm start
Install Axios for API requests:
npm install axios react-router-dom
Fetch data from the backend (src/App.js):
import React, { useEffect, useState } from ‘react’;
import axios from ‘axios’;
function App() {
const [users, setUsers] = useState([]);
useEffect(() => {
axios.get(‘http://localhost:5000/users’)
.then(res => setUsers(res.data))
.catch(err => console.log(err));
}, []);
return (
User List
{users.map(user => (
{user.name}
))}
);
}
export default App;
5. Connect Frontend to Backend
Modify the Express server to include a route for fetching users:
const User = require(‘./models/User’);
app.get(‘/users’, async (req, res) => {
const users = await User.find();
res.json(users);
});
Now, React can fetch data from the backend, completing the full-stack setup.
Conclusion
The MERN stack offers an efficient way to develop full-stack applications using JavaScript. With MongoDB for data storage, Express.js for server-side logic, React.js for the frontend, and Node.js as the backend runtime, developers can build dynamic and scalable web applications with ease.
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#MERN #NodeJS #ReactJS #MongoDB #ExpressJS #FullStackDevelopment #WebDevelopment #JavaScript