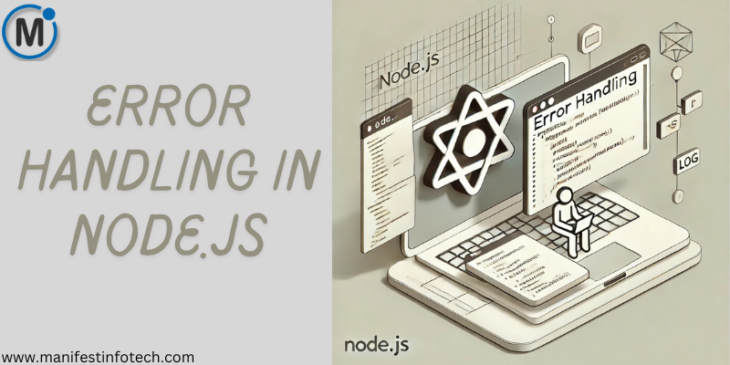
Error handling is a crucial part of building robust and maintainable applications in Node.js. Proper error management ensures a smooth user experience and helps developers debug and fix issues efficiently. This guide explores the best practices for handling errors in a Node.js application, including using try/catch blocks, custom error handling middleware, and logging errors.
1. Understanding Error Types in Node.js
Node.js errors can be categorized into:
Operational Errors: These are runtime errors that are expected and should be handled gracefully (e.g., invalid input, network failures, database connection issues).
Programmer Errors: These occur due to bugs in the code (e.g., undefined variables, null references, type errors). These should be fixed during development rather than handled at runtime.
2. Using Try/Catch for Synchronous Errors
For synchronous code, wrapping operations inside a try/catch block ensures that errors are caught and handled appropriately.
try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error(‘An error occurred:’, error.message);
}
3. Handling Asynchronous Errors
Since Node.js relies heavily on asynchronous operations, error handling requires special techniques:
Using Try/Catch in Async/Await
async function fetchData() {
try {
let data = await fetchSomeData();
console.log(data);
} catch (error) {
console.error(‘Error fetching data:’, error.message);
}
}
Handling Errors in Callbacks
For callback-based functions, error handling follows the standard pattern where the first argument in a callback represents an error:
fs.readFile(‘file.txt’, ‘utf8’, (error, data) => {
if (error) {
console.error(‘Error reading file:’, error.message);
return;
}
console.log(data);
});
4. Implementing Custom Error Classes
Custom error classes help differentiate between different types of errors.
class CustomError extends Error {
constructor(message, statusCode) {
super(message);
this.statusCode = statusCode;
}
}
throw new CustomError(‘Resource not found’, 404);
5. Using Express Error Handling Middleware
In an Express.js application, custom error-handling middleware ensures all errors are caught and handled consistently.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(err.statusCode || 500).json({ message: err.message || ‘Internal Server Error’ });
});
6. Logging Errors for Debugging
Logging errors helps in debugging and monitoring application performance. Use libraries like winston or pino for structured logging.
const winston = require(‘winston’);
const logger = winston.createLogger({
level: ‘error’,
transports: [new winston.transports.File({ filename: ‘error.log’ })],
});
app.use((err, req, res, next) => {
logger.error(err.message);
res.status(500).json({ error: ‘Something went wrong!’ });
});
7. Avoiding Uncaught Exceptions and Unhandled Rejections
To prevent crashes from uncaught exceptions and unhandled promise rejections, use:
process.on(‘uncaughtException’, (error) => {
console.error(‘Uncaught Exception:’, error);
});
process.on(‘unhandledRejection’, (reason, promise) => {
console.error(‘Unhandled Rejection at:’, promise, ‘reason:’, reason);
});
Conclusion
Effective error handling in Node.js improves application stability and security. By using structured error handling techniques such as try/catch, custom error classes, logging, and Express middleware, developers can create resilient applications that are easy to debug and maintain.
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#NodeJS #ErrorHandling #JavaScript #WebDevelopment #BackendDevelopment #Coding #SoftwareEngineering #ExpressJS #Logging #AsyncAwait #TechBlog