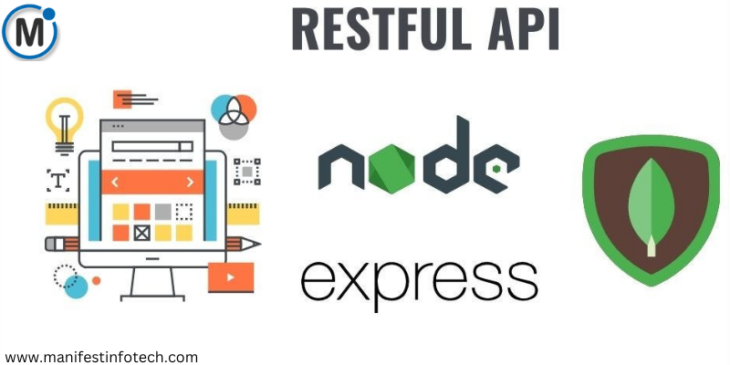
Building a RESTful API is a fundamental aspect of modern web development, enabling seamless communication between clients and servers. Node.js, known for its speed and scalability, is a popular choice for developing APIs. When combined with TypeScript, it enhances the development experience by introducing static typing, improved code maintainability, and better tooling support. In this blog, we will explore how to build a RESTful API using Node.js and TypeScript, highlighting the benefits of using TypeScript in a Node.js application.
Why Use TypeScript with Node.js?
TypeScript is a superset of JavaScript that introduces static typing, making code more predictable and reducing runtime errors. Here are some key benefits of using TypeScript with Node.js:
Type Safety: Detects errors at compile time, reducing unexpected runtime issues.
Better Code Maintainability: Encourages clean and structured code, making it easier to scale and maintain.
Improved Developer Experience: Provides better IntelliSense support in IDEs, enabling auto-completion and enhanced code navigation.
Object-Oriented Features: Supports interfaces, generics, and other object-oriented programming paradigms.
Seamless Integration with Modern Tooling: Works well with popular build tools, linters, and testing frameworks.
Setting Up a Node.js and TypeScript Project
Step 1: Initialize the Project
Start by creating a new Node.js project and setting up TypeScript.
mkdir node-typescript-api && cd node-typescript-api
npm init -y
Step 2: Install Required Dependencies
Install TypeScript and essential development dependencies.
npm install express cors dotenv
npm install –save-dev typescript ts-node @types/node @types/express @types/cors
Step 3: Configure TypeScript
Create a tsconfig.json file in the root directory:
{
“compilerOptions”: {
“target”: “ES6”,
“module”: “CommonJS”,
“outDir”: “./dist”,
“rootDir”: “./src”,
“strict”: true
}
}
Creating the RESTful API
Step 1: Create the Entry File
Inside the src directory, create an index.ts file.
import express, { Request, Response } from “express”;
import dotenv from “dotenv”;
import cors from “cors”;
dotenv.config();
const app = express();
const PORT = process.env.PORT || 5000;
app.use(cors());
app.use(express.json());
app.get(“/”, (req: Request, res: Response) => {
res.send(“Welcome to the TypeScript REST API!”);
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Step 2: Define Routes
Create a routes directory inside src and add a userRoutes.ts file.
import { Router } from “express”;
const router = Router();
router.get(“/users”, (req, res) => {
res.json({ message: “Fetching users…” });
});
export default router;
Modify index.ts to use this route:
import userRoutes from “./routes/userRoutes”;
app.use(“/api”, userRoutes);
Step 3: Run the Server
Modify package.json to include a start script:
“scripts”: {
“start”: “ts-node src/index.ts”
}
Run the API server:
npm start
Conclusion
Using TypeScript with Node.js for building a RESTful API enhances development by providing type safety, better tooling, and improved maintainability. In this guide, we set up a Node.js project with TypeScript, created a basic API, and implemented routing. This serves as a solid foundation for building scalable and robust APIs.
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#NodeJS #TypeScript #RESTAPI #WebDevelopment #BackendDevelopment #APIDevelopment #Coding #JavaScript #SoftwareEngineering #FullStack #WebDev #Tech #Programming #ExpressJS #TypeSafety #Developer #Code