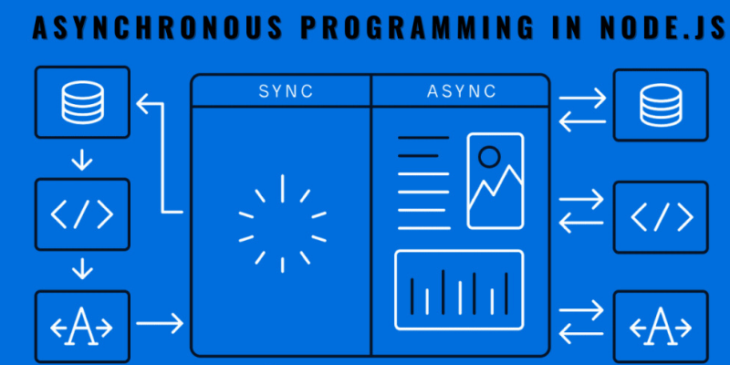
Asynchronous programming is a cornerstone of Node.js, enabling it to handle numerous operations simultaneously without blocking the execution of other code. This efficiency makes Node.js a popular choice for building scalable, high-performance applications, such as real-time web apps, chat servers, and APIs. To understand how asynchronous programming works in Node.js, let’s explore its three primary mechanisms: callbacks, promises, and async/await.
Callbacks: The Foundation of Asynchronous Programming
A callback is a function passed as an argument to another function and is invoked after the completion of a specific task. Callbacks are the simplest way to manage asynchronous operations in Node.js. For instance:
const fs = require(‘fs’); fs.readFile(‘example.txt’, ‘utf-8’, (err, data) => { if (err) { console.error(‘Error reading file:’, err); return; } console.log(‘File content:’, data); });
In the example above, the readFile
method reads a file asynchronously. The callback function is executed once the file is read, handling errors and processing the file’s content.
While callbacks are straightforward, they can lead to “callback hell” — a situation where nested callbacks make the code difficult to read and maintain. For example:
doSomething((err, result) => { if (err) return handleError(err); doSomethingElse(result, (err, newResult) => { if (err) return handleError(err); doThirdThing(newResult, (err, finalResult) => { if (err) return handleError(err); console.log(‘Final result:’, finalResult); }); }); });
Promises: A Cleaner Alternative
Promises address the issues of callback hell by providing a cleaner and more structured way to handle asynchronous operations. A promise represents a value that may be available now, or in the future, or never. Promises have three states: pending, fulfilled, and rejected.
Here’s how the file-reading example looks using promises:
const fs = require(‘fs’).promises; fs.readFile(‘example.txt’, ‘utf-8’) .then(data => { console.log(‘File content:’, data); }) .catch(err => { console.error(‘Error reading file:’, err); });
Using promises, we chain .then()
for success and .catch()
for error handling. Promises make the flow of asynchronous code more readable and maintainable.
Async/Await: Synchronous Style for Asynchronous Code
Introduced in ECMAScript 2017, async/await builds on promises to provide a syntax that resembles synchronous code. This makes it easier to write and debug asynchronous operations.
To use async/await, declare a function as async and use the await keyword before a promise. Here’s the file-reading example with async/await:
const fs = require(‘fs’).promises; async function readFileAsync() { try { const data = await fs.readFile(‘example.txt’, ‘utf-8’); console.log(‘File content:’, data); } catch (err) { console.error(‘Error reading file:’, err); } } readFileAsync();
Async/await makes the code appear linear and eliminates the need for multiple .then()
calls. It also integrates seamlessly with try/catch
for error handling, making it more intuitive for developers.
Comparing the Three Approaches
- Callbacks: Simple but can lead to deeply nested and hard-to-read code.
- Promises: Cleaner than callbacks and offers chaining for sequential tasks.
- Async/Await: The most modern and readable approach, ideal for complex workflows.
When to Use What?
- Use callbacks for simple operations or when working with legacy codebases.
- Use promises when you need better readability and chaining but don’t require the synchronous-like style of async/await.
- Use async/await for new projects or when you need to manage multiple asynchronous operations in a clean and structured way.
Conclusion
Asynchronous programming is essential for unlocking Node.js’s true potential. While callbacks laid the foundation, promises and async/await have significantly improved code readability and maintainability. By mastering these techniques, developers can create robust, efficient, and scalable Node.js applications capable of handling real-world demands with ease.
If you are looking for any services related to Website Development, App Development, Digital Marketing and SEO, just email us at nchouksey@manifestinfotech.com or Skype id: live:76bad32bff24d30d
𝐅𝐨𝐥𝐥𝐨𝐰 𝐔𝐬:
𝐋𝐢𝐧𝐤𝐞𝐝𝐢𝐧: linkedin.com/company/manifestinfotech
𝐅𝐚𝐜𝐞𝐛𝐨𝐨𝐤: facebook.com/manifestinfotech/
𝐈𝐧𝐬𝐭𝐚𝐠𝐫𝐚𝐦: instagram.com/manifestinfotech/
𝐓𝐰𝐢𝐭𝐭𝐞𝐫: twitter.com/Manifest_info
#NodeJS #AsynchronousProgramming #JavaScript #Callbacks #Promises #AsyncAwait #WebDevelopment #BackendDevelopment #NodeJSTips #CodingBestPractices #SoftwareEngineering #WebAppDevelopment #Scalability #FullStackDevelopment #TechTips